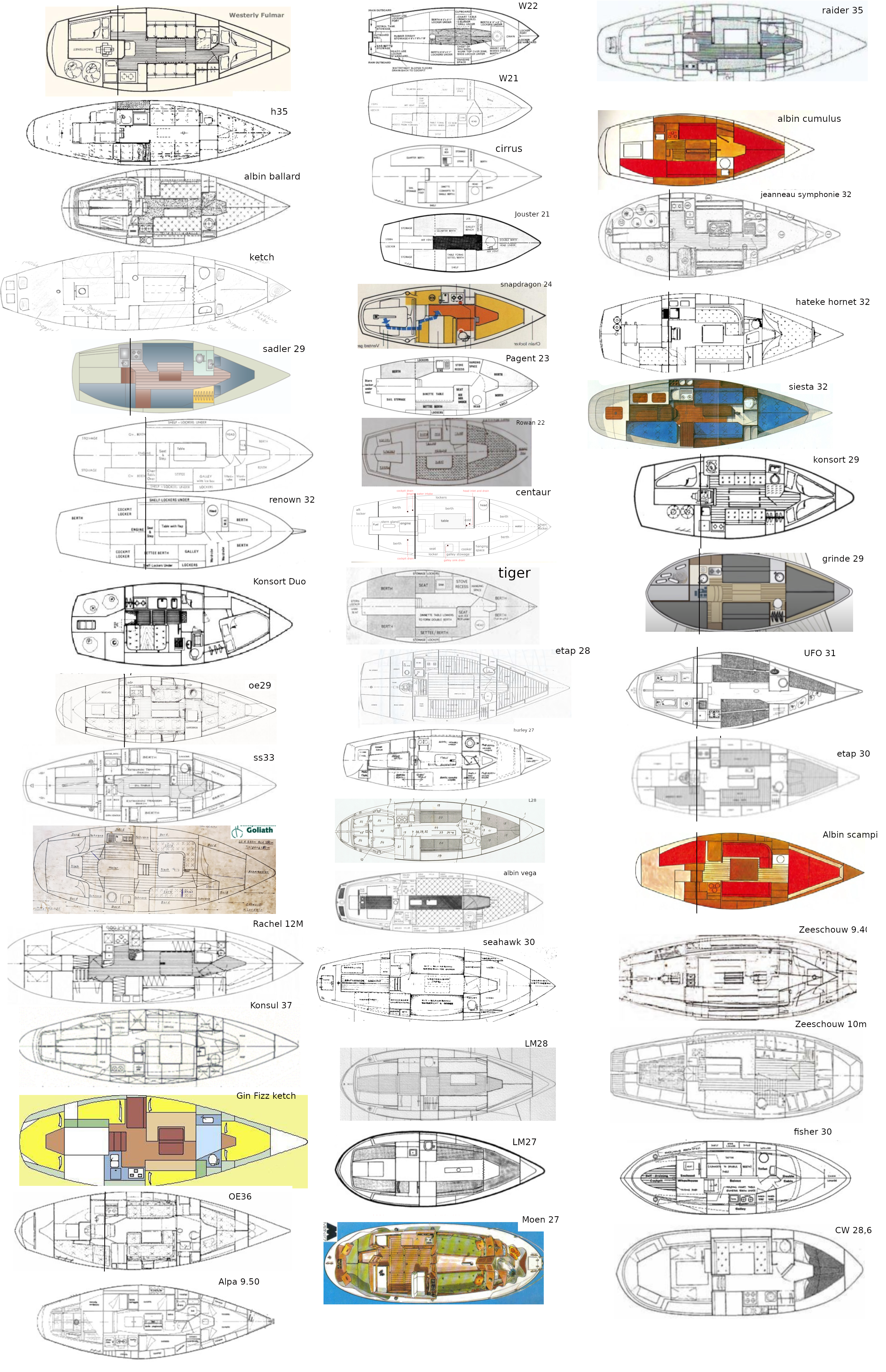
Comparing affordable sailboats by their interior size
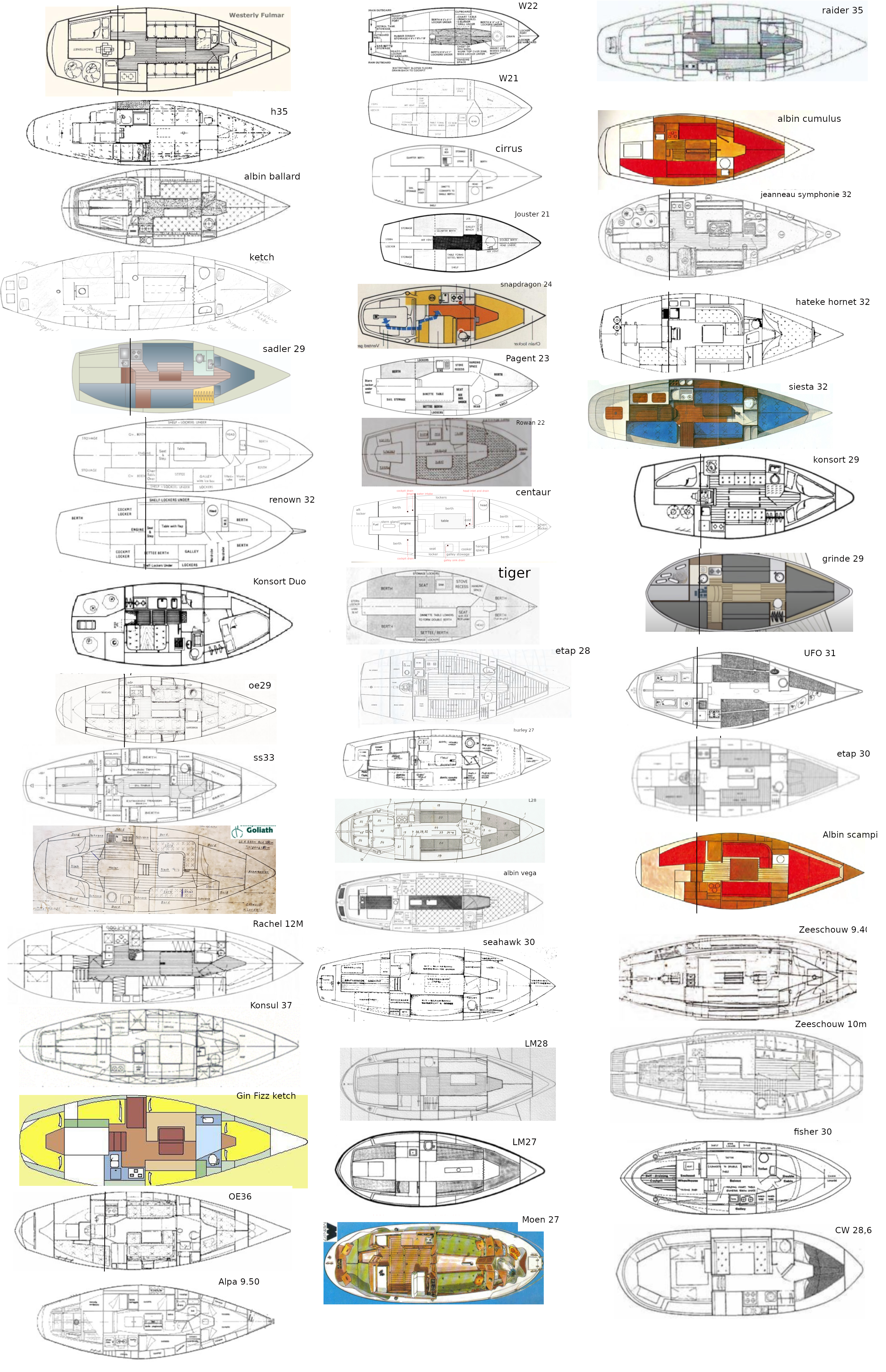
First make sure the DrawCentered function is called after the image loads as below
<div>
<ul>
<li>
<img src="exampleimage.png" onload="DrawCentered(this);"/>
</li>
</ul>
</div>
Then add the resizing code below to your html file (for example: in document load function). The images will allways be centered within the container DIV, regardless of image size or page width.
window.onresize = function() {
$( "li img" ).each(function( index ) {
DrawCentered(this);
});
}
function DrawCentered(im)
{
var gScaledHeight, gScaledWidth;
var iWidth=im.width, iHeight=im.height;
var cWidth=$(im).closest("div").width();
var cHeight=$(im).closest("div").height();
gScaledWidth = iWidth*(cHeight/iHeight);
// see which is the image's biggest dimension
if( cWidth > gScaledWidth ) //scale cHeight
{
// ratio of new to old cWidth
gScale = gScaledWidth/iWidth;
gScaledHeight = gScale*iHeight;
gOffY = 0;
gOffX = (cWidth-gScaledWidth)/2.0
}
else
{
gScaledHeight = iHeight*(cWidth/iWidth);
gScale = gScaledHeight/iHeight;
gScaledWidth = gScale*iWidth;
gOffX = 0;
gOffY = (cHeight-gScaledHeight)/2.0;
}
$(im).css("width",gScaledWidth);
$(im).css("height",gScaledHeight);
$(im).css("padding-top",gOffY+"px");
$(im).css("padding-left",gOffX+"px");
}
Here is a link to my new Twitter bookmarklet: https://ejectamenta.com/bookmarklets/twitter-de-propandarizer-bookmarklet/
Use it to get rid of the panel on the right in Twitter, the panel with all the mainstream news propaganda on it.
Drag it to your bookmarks bar and click it go get rid of the panel.
Enhance your twitter experience by deleting the bullshit!
Here are some of the 3D models I have made, mostly in HeeksCAD as its quick and easy, for the camera track, ladder foot & lens cap I used FreeCAD for its extra features. You can rotate and zoom the models in the 3D view below.
If you find the models useful then please consider donating using the paypal button
This is a design I made of a negative holder for analogue camera film, I use it with my digital camera to scan negatives. You can see an example of how I use the negative holder here
You can download it here
This is a design I made for a bellows, it’s the track for a Kenlock “Swing & Shift” tilt shift bellows. Made from ABS it seemed to be more robust than the original hard plastic track.
You can download it here
This is a design I made for a cranking tool for a IRUS U600 single axis tractor, it takes a M8 bolt that must be then modified to be gripped by a suitable drill chuck.
You can download it here
I also made a stronger one here, haven’t tested it yet though, maybe it doesn’t fit. You can download it here
This is a shower holder, I threw out my old showerhead and forgot that it had the mount attached, I decided to knock one up.
You can download it here
This is a vacuum adapter for a mitre circular saw, the old one was pretty brittle and broke.
You can download it here
This is a lens cap for a Zenit MIR KMZ 20M lens, Due to the size of the lens and the protruding glass its a bit difficult to find a suitable lens cap, so I made one, it’s a push fit.
You can download it here
This is a design I made for a compact asthma inhaler body. It should be adjusted since it doesn’t allow the canister to fit inside the body. Also the nozzle didn’t really work as the 3D printing wasn’t capable of such small holes. It probably needs a microdrill to finish.
You can download it here
This is ladder foot. They are a bit difficult to find in the shops.
You can download it here
I’ve tested all my vintage M42, Tamron Adaptall and Minolta/Sony lenses and produced a web page showing the results. The results can be seen at the following page 👇
The big lens test
This is some code for building a jquery ui horizontal menu. It is based on code by https://codepen.io/seungjaeryanlee/ and modified for icon support and to create a menu automatically from a JSON schema. It’s pretty useful for HTML5 app development.
This is how the menu would look be structured if just coded in html
<ul id="menubar">
<li><div class="icon"><span class="ui-icon ui-icon-folder-open"></span><a href="#">Alpha</a></div>
<ul>
<li><div><a href="#">Beta 1</a></div></li>
<li>
<div class="icon"><span class="ui-icon ui-icon-folder-open"></span><a href="#">Beta 2</a></div>
<ul>
<li><div class="icon"><span class="ui-icon ui-icon-folder-open"></span><a href="#">Beta 2a</a></div></li>
<li><div><a href="#">Beta 2b</a></div></li>
</ul>
</li>
<li><div><a href="#">Beta 3</a></div></li>
</ul>
</li>
<li>
<div><a href="#">Beta</a></div>
<ul>
<li><div><a href="#">Beta 1</a></div></li>
<li>
<div><a href="#">Beta 2</a></div>
<ul>
<li><div><a href="#">Beta 2a</a></div></li>
<li><div><a href="#">Beta 2b</a></div></li>
</ul>
</li>
<li><div><a href="#">Beta 3</a></div></li>
</ul>
</li>
<li><div><a href="#">Gamma</a></div></li>
<li><div><a href="#">Delta</a></div></li>
</ul>
menuStructure is the JSON menu descriptor, the code setUpMenuHTML and addMenuHTML create HTML from this schema in the format of the html above.
var menuStructure = {menu: [
{ name: "file",
menu: [
{name: "Open", icon: "ui-icon ui-icon-folder-open"},
{name: "save", icon: "ui-icon ui-icon-disk"}
]
},
{name: "ImageProcessing", icon: "ui-icon ui-icon-image",
menu: [
{name: "Invert"},
{name: "threshold"},
{name: "binary",
menu: [
{name: "erode"},
{name: "open"},
{name: "close"}
]
}
]
},
{name: "help", icon: "ui-icon ui-icon-help"}
]};
function addMenuHTML(ME, $selector)
{
var $ulOuter = $selector;
if(!$selector.is('#menubar'))
$ulOuter = $("
").appendTo($selector);
for(var j=0;j").appendTo($ulOuter);
if(ME.menu[j].icon)
{
str = ''+ME.menu[j].name+'';
}
else{
str = '' + ME.menu[j].name + '';
}
$(str).appendTo($liInner);
// call recursively on nested menu elements
if(ME.menu[j].menu)
addMenuHTML(ME.menu[j], $liInner);
}
}
function setUpMenuHTML()
{
var $menu = $('#menubar');
$menu.empty();
addMenuHTML(menuStructure, $menu);
}
function setUpMenu()
{
$('#menubar').menu();
$('#menubar').menu({
position: { my: 'left top', at: 'left bottom' },
blur: function() {
$(this).menu('option', 'position', { my: 'left top', at: 'left bottom' });
},
focus: function(e, ui) {
if ($('#menubar').get(0) !== $(ui).get(0).item.parent().get(0)) {
$(this).menu('option', 'position', { my: 'left top', at: 'right top' });
}
},
});
}
$(document).ready(function() {
setUpMenuHTML();
setUpMenu();
});
the CSS ensures that the menuu is horizonal and that the icons are correctly displayed next to the menu names and that the sub menus also indent correctly
#menubar {
position: fixed;
top: 0;
left: 0;
width: 100%;
}
ul > li a{
max-width:100%;
color: inherit; /* blue colors for links too */
text-decoration: inherit; /* no underline */
}
li > div {
white-space: nowrap;
overflow: hidden;
}
/* Make jQuery UI Menu into a horizontal menubar with vertical dropdown */
#menubar > li { /* Menubar buttons */
#text-align: center;
display: inline-block;
}
#menubar li > div:not(.icon) {
padding-left: 0.3em !important;
}
#menubar li > div.icon {
padding-left: 1.5em;
}
#menubar > li > ul > li { /* Menubar buttons inside dropdown */
display: block;
}
/* Change dropdown carets to correct direction */
#menubar > li > div > span.ui-icon-caret-1-e {
/* Caret on menubar */
background:url(https://www.drupal.org/files/issues/ui-icons-222222-256x240.png) no-repeat -64px -16px !important;
}
#menubar ul li div span.ui-icon-caret-1-e {
/* Caret on dropdowns */
background:url(https://www.drupal.org/files/issues/ui-icons-222222-256x240.png) no-repeat -32px -16px !important;
}
This website contains the free online HTML5 puzzle games: MegaTangram (a tangram game), hexpac (a hexominos packing game), Enigmatic puzzle, befuddled and PegSolitare. They work great with any modern browser, However they are sometimes being worked on so may have temporary glitches or present new features from time to time.
The website is currently being migrated from a CMSMS website, the two CMS systems are quite different, I’m still working on implementing the functionality and some things may not work correctly.
Hope you enjoy it
Cheers
Dave
Now main user login, user home page and puzzle pages are secured by SSL/https system. Not all pages are using https however, so check when using the quick login fields by the menu bar if you want to be extra secure. Using https is needed for facebook apps so hopefully the puzzles will soon be available on Facebook as well.
The RhythmSticks app is a simulation of a 5 piece drum kit using quality sampled drum sounds. The sounds have 6 zones of loudness which are increased by hitting the drum near the center (or at the edge for the cymbals). The hihat has partially and fully open sounds and a foot splash or tap, The 24 inch ride cymbal has additional bell sounds. Cymbals are hihat, 18 & 16 inch crashes, 18 & 24 inch rides, 6 inch splash and a 22 inch china.
Click the metronome and time is started now when you play the drums the score reflects the drums hit and the rhythm played is recorded. To play your recorded score click again on the metronome, you can even add extra beats as it is is being replayed.
The mouse may not be the best device to play the drums with and by entering the menu (top left button) the keyboard keys can be mapped to the drums
The WebAudio API used for this app is pretty new technology so you will need a recent browser for the app to work, on a tablet you will probably need a recent model and the latest browser (ie. chrome beta).
Also available on facebook
For more information on browser support see: Can I use Web Audio API